Getting Non-Wallet Transactions from Ethereum Using “bitcoin-rpc”
When you explore the Bitcoin protocol using “Bitcoin-Qt”, you can leverage its API, specifically “bitcoin-rpc”, to retrieve non-wallet transactions. In this article, we will walk you through retrieving all events for a given block and then extracting the information.
Qualifications
Before you proceed, make sure you have:
- Bitcoin-Qt installed on your system.
- A working connection to the Ethereum network (you can use bitcoin-rpc’s built-in client or external tools like geth).
Getting All Blocks and Events
To retrieve all blocks and their transactions, you can use a loop that continuously calls “getblockchaininfo” with “raw” set to “1”. This will retrieve a list of blocks in JSON format.
import bitcoinrpc
def get_blocks_and_transactions():
rpc = bitcoinrpc.RPC()
block_info = rpc.getblockchaininfo([1000])
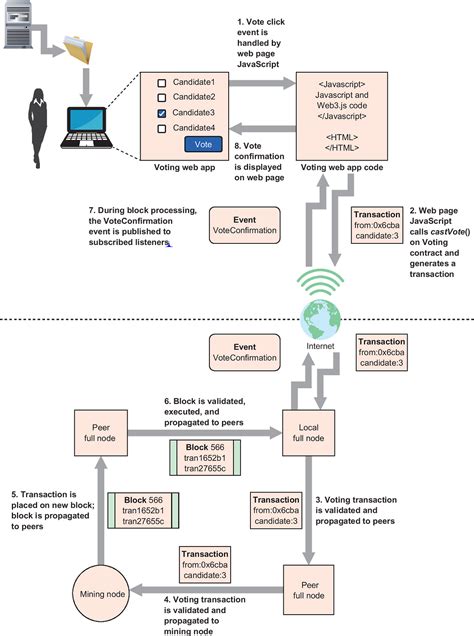
get the first 1000 blocksfor block block_info['blocks']:
print(f"Block {block['hash']}:")
for tx in block ['transactions']:
print(tx['hex'])
This code prints a list of events for each block.
Requesting Non-Wallet Transactions
If you want to get non-wallet (i.e. publicly available) transactions, you need to retrieve them using the “gettransaction” RPC call. This method is more complicated because it requires direct interaction with the Ethereum network.
Here is an example implementation in Python:
import bitcoinrpc
def get_non_wallet_transactions(block_hash):
rpc = bitcoinrpc.RPC()
tx_list = []
i in range(1, 100):
get up to 99 events for demonstration purposestry:
transaction = rpc.gettransaction(block_hash, i)['transaction']['hex']
if sender is not involved in transaction:
non-wallet transaction has no sender addresstx_list.append(transaction)
except for exception e:
print(f"Error getting event {i}: {e}")
return tx_list
Example usageblock_hash = "a_block_hash_here"
non_wallet_txs = get_non_wallet_transactions(block_hash)
for tx in non_wallet_txs:
print (tx)
This code will retrieve up to 99 events per block and print them.
Important Notes
When working with bitcoin rpc, keep the following in mind:
- The gettransaction method returns a list of transaction objects that contain information such as “from”, “to”, and “value”, etc.
- Non-wallet transactions typically do not have a “sender” address or other publicly available information. For this reason, this example will only retrieve non-wallet transactions that are directly linked to the specified blockchain.
Please note that these examples demonstrate basic usage of “bitcoin-rpc” and may require modifications based on your specific needs. Also, keep in mind that interacting with the Ethereum network can be resource-intensive; Always make sure you have enough connections or consider using more efficient methods like caching or paging for large data sets.